Dataframes
Rerun, at its core, is a database. As such, you can always get your data back in the form of tables (also known as dataframes, or records, or batches...).
This can be achieved in three different ways, depending on your needs:
- using the dataframe API, currently available in Python and Rust,
- using the blueprint API to configure a dataframe view from code,
- or simply by setting up dataframe view manually in the UI.
This page is meant as a reference to get you up and running with these different solutions as quickly as possible. For an in-depth introduction to the dataframe API and the possible workflows it enables, check out our Getting Started guide or one of the accompanying How-Tos.
We'll need an RRD file to query. Either use one of yours, or grab some of the example ones, e.g.:
curl 'https://app.rerun.io/version/latest/examples/dna.rrd' -o - > /tmp/dna.rrd
Using the dataframe API using-the-dataframe-api
The following snippet demonstrates how to query the first 10 rows in a Rerun recording using latest-at (i.e. time-aligned) semantics:
"""Query and display the first 10 rows of a recording."""
import sys
import rerun as rr
path_to_rrd = sys.argv[1]
recording = rr.dataframe.load_recording(path_to_rrd)
view = recording.view(index="log_time", contents="/**")
batches = view.select()
for _ in range(10):
row = batches.read_next_batch()
if row is None:
break
# Each row is a `RecordBatch`, which can be easily passed around across different data ecosystems.
print(row)
Check out the API reference to learn more about all the ways that data can be searched and filtered:
Using the blueprint API to configure a dataframe view using-the-blueprint-api-to-configure-a-dataframe-view
The following snippet demonstrates how visualize an entire Rerun recording using latest-at (i.e. time-aligned) semantics by displaying the results in a dataframe view:
"""Query and display the first 10 rows of a recording in a dataframe view."""
import sys
import rerun as rr
import rerun.blueprint as rrb
path_to_rrd = sys.argv[1]
rr.init("rerun_example_dataframe_view_query", spawn=True)
rr.log_file_from_path(path_to_rrd)
blueprint = rrb.Blueprint(
rrb.DataframeView(
origin="/",
query=rrb.archetypes.DataframeQuery(
timeline="log_time",
apply_latest_at=True,
),
),
)
rr.send_blueprint(blueprint)
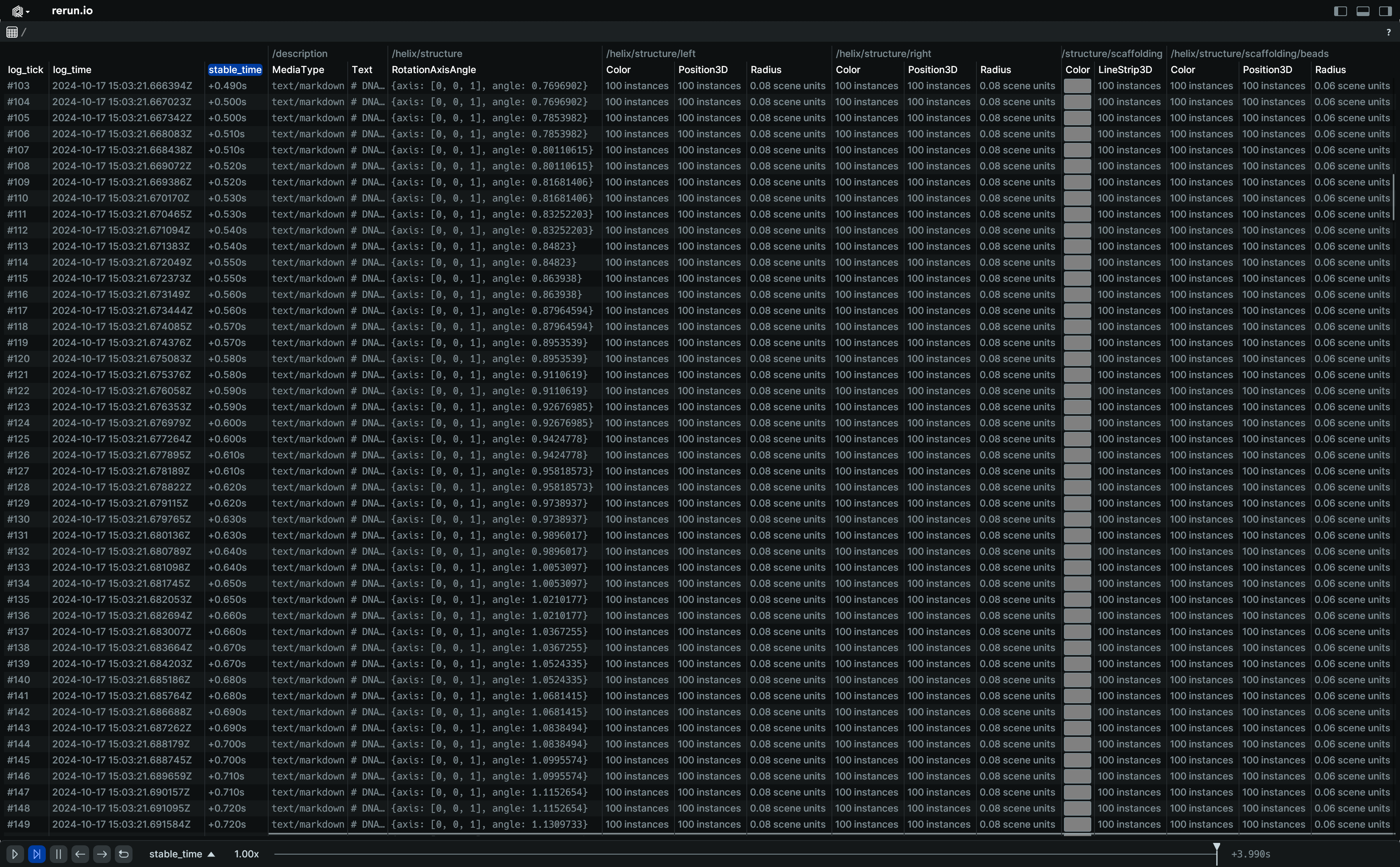
Aside: re-using blueprint files from other SDKs
While the blueprint APIs are currently only available through Python, blueprints can be saved and re-logged as needed from any language our SDKs support.
First, save the blueprint to a file (.rbl
by convention) using either the viewer (Menu > Save blueprint
) or the python API:
"""Craft a blueprint with the python API and save it to a file for future use."""
import sys
import rerun.blueprint as rrb
path_to_rbl = sys.argv[1]
rrb.Blueprint(
rrb.DataframeView(
origin="/",
query=rrb.archetypes.DataframeQuery(
timeline="log_time",
apply_latest_at=True,
),
),
).save("rerun_example_dataframe_view_query", path_to_rbl)
Then log that blueprint file in addition to the data itself:
"""
Query and display the first 10 rows of a recording in a dataframe view.
The blueprint is being loaded from an existing blueprint recording file.
"""
# python dataframe_view_query_external.py /tmp/dna.rrd /tmp/dna.rbl
import sys
import rerun as rr
path_to_rrd = sys.argv[1]
path_to_rbl = sys.argv[2]
rr.init("rerun_example_dataframe_view_query_external", spawn=True)
rr.log_file_from_path(path_to_rrd)
rr.log_file_from_path(path_to_rbl)
Check out the blueprint API and log_file_from_path
references to learn more:
- 🐍 Python blueprint API reference
- 🐍 Python
log_file_from_path
- 🦀 Rust
log_file_from_path
- 🌊 C++
log_file_from_path
You can learn more in our dedicated page about blueprint re-use.
Setting up dataframe view manually in the UI setting-up-dataframe-view-manually-in-the-ui
The same dataframe view shown above can be configured purely from the UI: