Query semantics & partial updates
The Rerun data model is based around streams of entities with components the-rerun-data-model-is-based-around-streams-of-entities-with-components
In Rerun, you model your data using entities (roughly objects) with batches of components that change over time.
An entity is identified by an entity path, e.g. /car/lidar/points
, where the path syntax can be used to model hierarchies of entities.
A point cloud could be made up of positions and colors, but you can add whatever components you like to the entity.
Point positions are e.g. represented as a batch of Position3D
component instances.
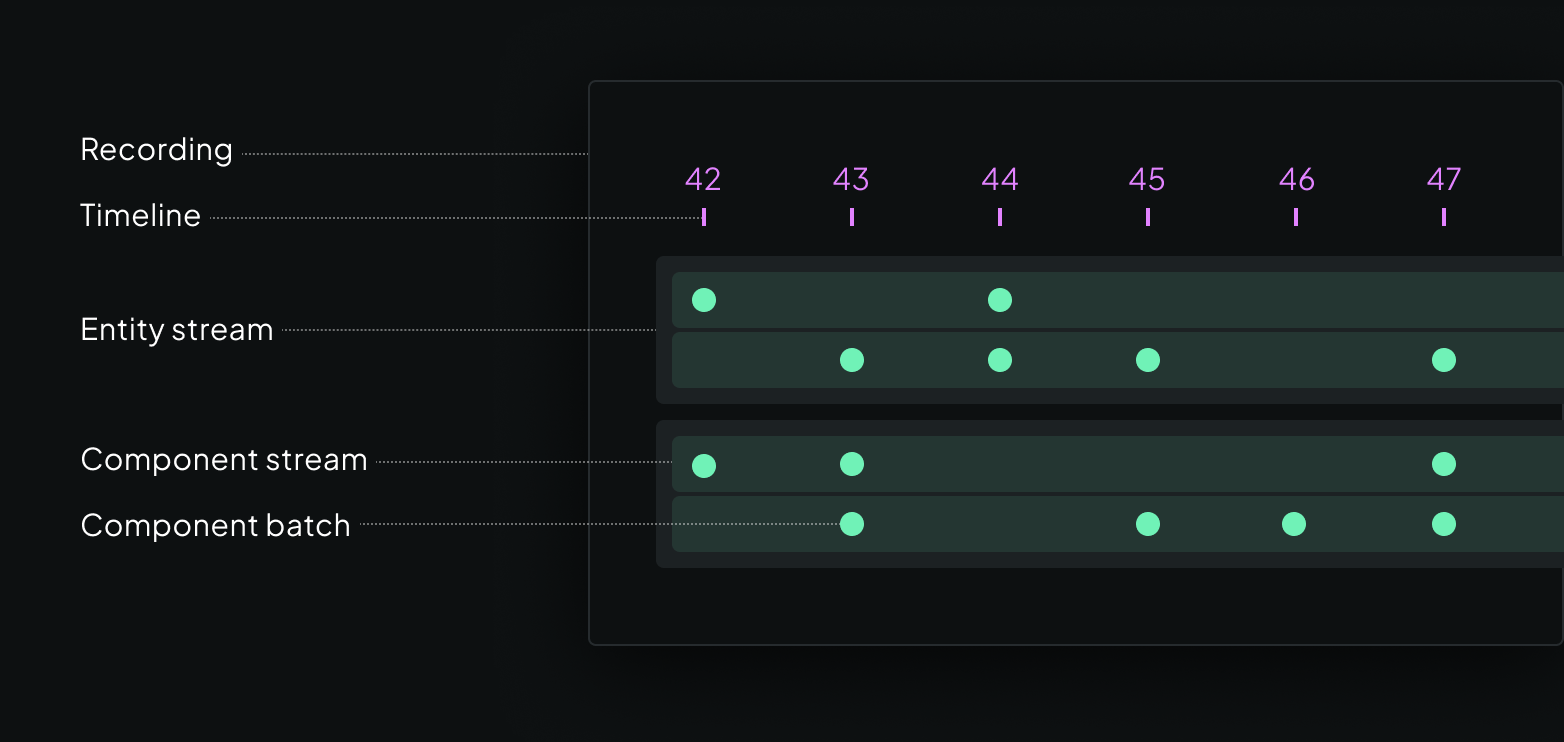
Components can have different values for different times, and do not have to be updated all at once. Rerun supports multiple timelines (sequences of times), so that you can explore your data organized according to e.g. the camera's frame index or the time it was logged.
Core queries core-queries
All data that gets sent to the Rerun viewer is stored in an in-memory database, and there are two core types of queries against the database that visualizers in the viewer run.
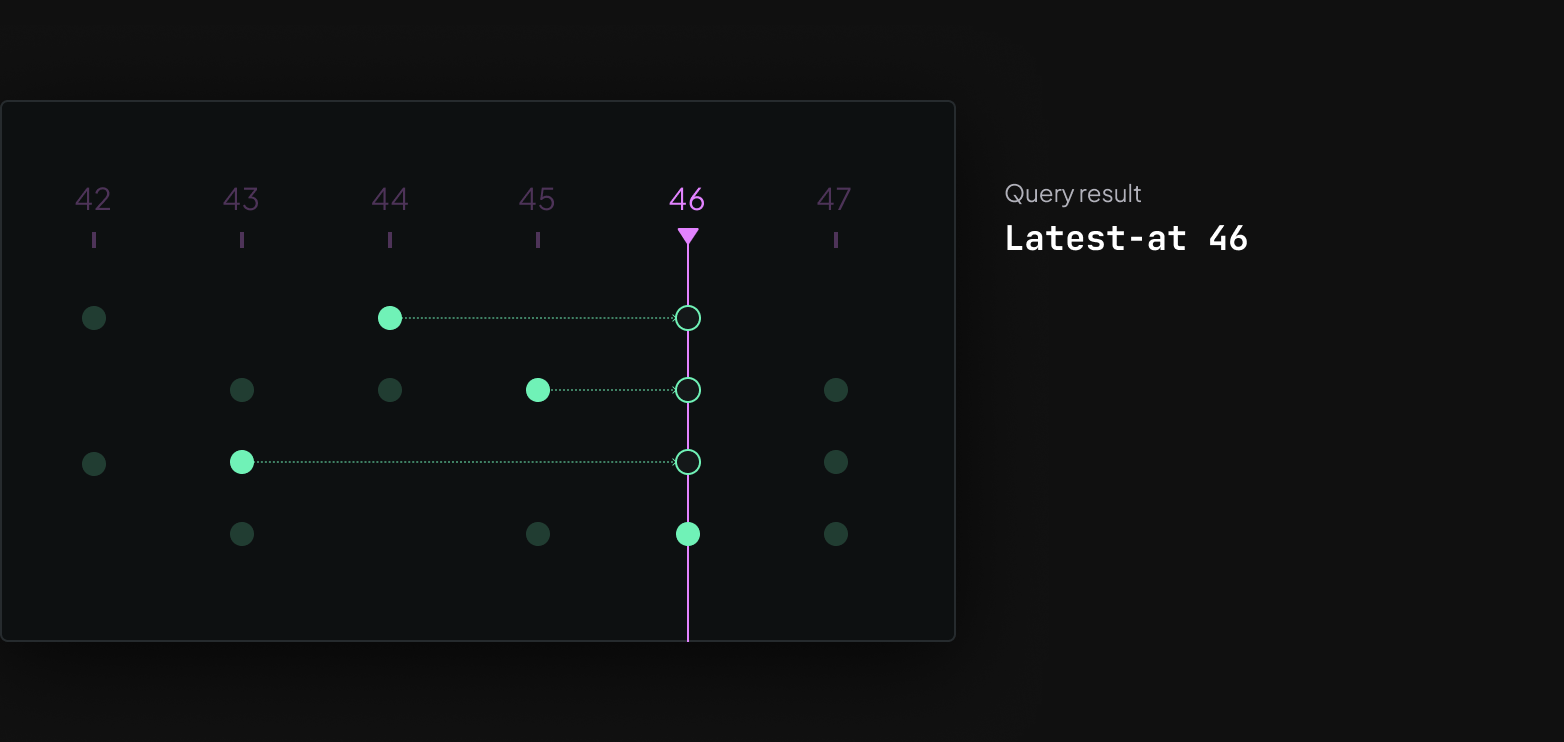
Latest-at queries collect the latest version of each of an entity's components at a particular time. This allows the visualizer to draw the current state of an object that was updated incrementally. For example, you might want to update the vertex positions of a mesh while keeping textures and triangle indices constant.
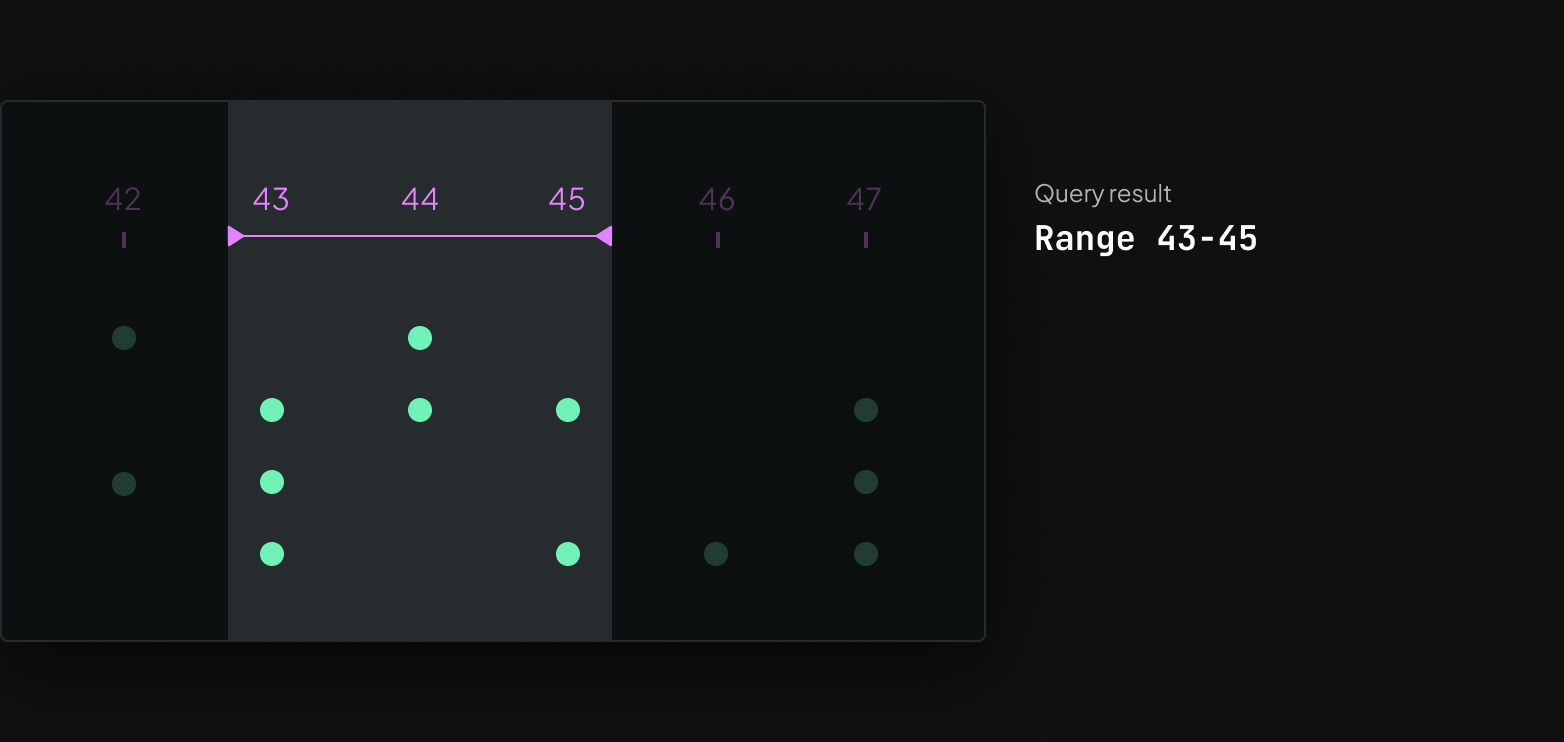
Range queries instead collect all components associated with times on a time range. These queries drive any visualization where data from more than one time is shown at the same time. The obvious example is time series plots, but it can also be used to e.g. show lidar point clouds from the last 10 frames together.
The queried range is typically configurable, see for instance this how-to guide on fixed windows plots for more information.
Partial updates partial-updates
As mentioned above, the query semantics that power the Rerun Viewer, coupled with our chunk-based storage, make it possible to log only the components that have changed in-between frames (or whatever atomic unit your timeline is using).
Here's an example of updating only some specific properties of a point cloud, over time:
"""Update specific properties of a point cloud over time."""
import rerun as rr
rr.init("rerun_example_points3d_partial_updates", spawn=True)
positions = [[i, 0, 0] for i in range(0, 10)]
rr.set_time_sequence("frame", 0)
rr.log("points", rr.Points3D(positions))
for i in range(0, 10):
colors = [[20, 200, 20] if n < i else [200, 20, 20] for n in range(0, 10)]
radii = [0.6 if n < i else 0.2 for n in range(0, 10)]
# Update only the colors and radii, leaving everything else as-is.
rr.set_time_sequence("frame", i)
rr.log("points", rr.Points3D.from_fields(radii=radii, colors=colors))
# Update the positions and radii, and clear everything else in the process.
rr.set_time_sequence("frame", 20)
rr.log("points", rr.Points3D.from_fields(clear_unset=True, positions=positions, radii=0.3))
To learn more about how to use our partial updates APIs, refer to this page.