Scalar
Log a double-precision scalar.
The current timeline value will be used for the time/X-axis, hence scalars cannot be timeless.
When used to produce a plot, this archetype is used to provide the data that
is referenced by the SeriesLine
or SeriesPoint
archetypes. You can do
this by logging both archetypes to the same path, or alternatively configuring
the plot-specific archetypes through the blueprint.
Components
Required: Scalar
Links
Examples
Simple line plot
"""Log a scalar over time.""" import math import rerun as rr rr.init("rerun_example_scalar", spawn=True) # Log the data on a timeline called "step". for step in range(0, 64): rr.set_time_sequence("step", step) rr.log("scalar", rr.Scalar(math.sin(step / 10.0)))
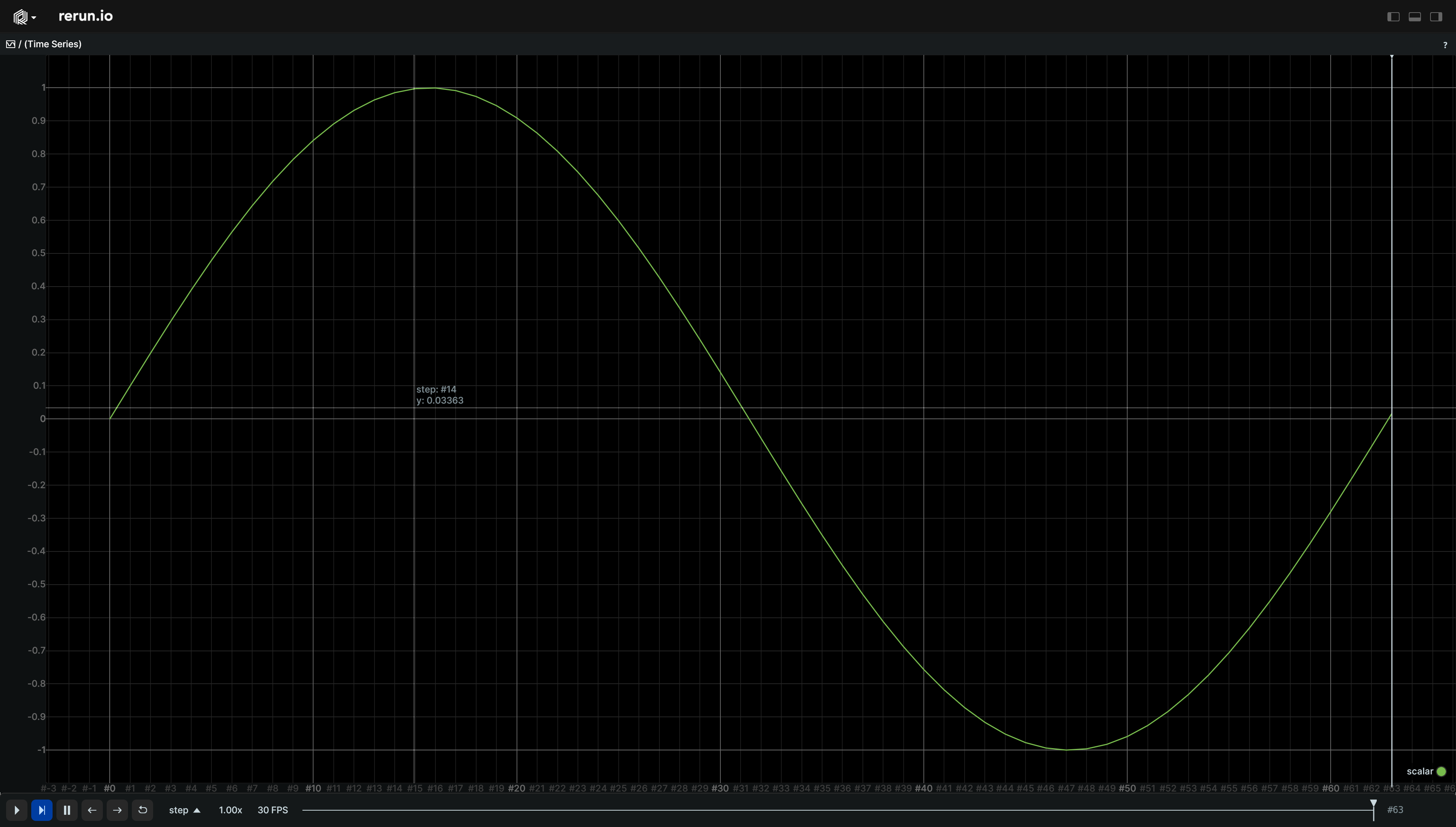
Multiple time series plots
"""Log a scalar over time.""" from math import cos, sin, tau import numpy as np import rerun as rr rr.init("rerun_example_scalar_multiple_plots", spawn=True) lcg_state = np.int64(0) # Set up plot styling: # They are logged timeless as they don't change over time and apply to all timelines. # Log two lines series under a shared root so that they show in the same plot by default. rr.log("trig/sin", rr.SeriesLine(color=[255, 0, 0], name="sin(0.01t)"), timeless=True) rr.log("trig/cos", rr.SeriesLine(color=[0, 255, 0], name="cos(0.01t)"), timeless=True) # Log scattered points under a different root so that they show in a different plot by default. rr.log("scatter/lcg", rr.SeriesPoint(), timeless=True) # Log the data on a timeline called "step". for t in range(0, int(tau * 2 * 100.0)): rr.set_time_sequence("step", t) rr.log("trig/sin", rr.Scalar(sin(float(t) / 100.0))) rr.log("trig/cos", rr.Scalar(cos(float(t) / 100.0))) lcg_state = (1140671485 * lcg_state + 128201163) % 16777216 # simple linear congruency generator rr.log("scatter/lcg", rr.Scalar(lcg_state))
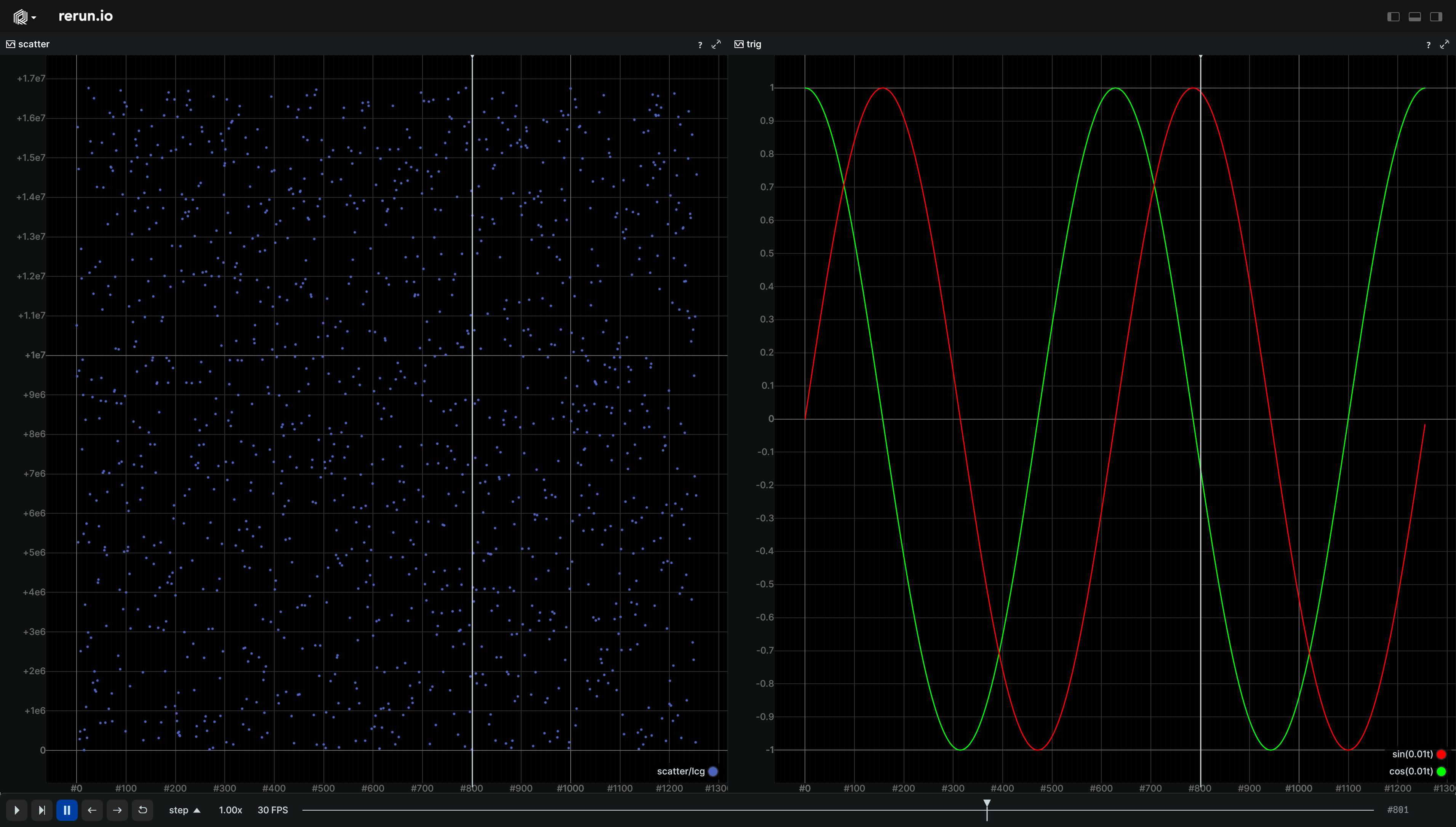